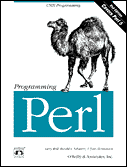
|
|
CGI Programming with Perl
Lesson 3: Operators
Precedence | Operation | Arithmetic | String |
1 | AutoIncrement | ++ | |
| AutoDecrement | -- | |
2 | negation | - | |
3 | Exponentiation | ** | |
4 | Multiplication | * | |
| Division | / | |
| Modulo | % | |
| Repetition | | x |
5 | Addition | + | |
| Subtraction | - | |
| Concatenation | | . |
6 | less than | < | lt |
| less than or equal to | <= | le |
| greater than | > | gt |
| greater than or equal to | >= | ge |
7 | equivalent | == | eq |
| not equivalent | != | ne |
| compare | <=> | cmp |
8 | NOT | ! | ! |
9 | AND | && | && |
10 | OR | || | || |
11 | Simple Assignment | = | = |
| Multiplication and assignment | *= | |
| Division and assignment | /= | |
| Addition and assignment | += | |
| Subtraction and assignment | -= | |
| Remainder and assignment | %= | |
| Exponentiation and assignment | **= | |
| Bitwise AND and assignment | &= | |
| Bitwise OR and assignment | |= | |
| Bitwise XOR and assignment | ^= | |
| String concatenation and assignment | | .= |
| String repeat and assignment | | x= |
12 | mnemonic NOT | not | not |
13 | mnemonic AND | and | and |
14 | mnemonic OR | or | or |
Exercises
1 |
#! e:\perl\bin\perl.exe
# ask the user for a temperature and then ask
# whether to convert to Celsius or Fahrenheit.
print "Celsius / Fahrenheit Conversion\n";
print "Enter known temperature: ";
$temp = <STDIN>;
chomp $temp;
print "Convert to [C]elsius or [F]ahrenheit: ";
$convert = <STDIN>;
chomp $convert;
if ($convert eq "C" || $convert eq "c") {
print $temp . "F = " . (($temp - 32) * 5/9) . "C";
} elsif ($convert eq "F" || $convert eq "f") {
print $temp . "C = " . (($temp * 9/5) + 32) . "F";
} else {
print "Unknown Conversion Type " . $convert;
}
#!C:\perl\bin\perl.exe
# Exercise 3.1
# Temperature conversion
# Get the temperature from the user
print("Enter a positive integer: ");
$temp = <STDIN>;
chomp($temp);
# Get the conversion type from the user
print("Convert to degrees F or C (F/C)? ");
$type = <STDIN>;
chomp($type);
if ($type eq "F") {
$T = 9 * $temp / 5 + 32;
} else {
$T = 5 * ($temp - 32) / 9;
}
print("$T degrees $type\n");
| |
2 |
#!C:\perl\bin\perl.exe
# Exercise 3.2
# Sort two strings
# Get the first string
print("Enter first string: ");
$string1 = <STDIN>;
# Get the second string
print("Enter second string: ");
$string2 = <STDIN>;
# Test and print the strings in sorted order
# Note: newline character left
# on the end of the strings
print(($string1 ge $string2) ? $string2 : $string1);
print(($string1 lt $string2) ? $string2 : $string1);
| |
fatpat Software · PO Box 1785 · Charlottesville, VA 22902 · (804) 977-1652
|